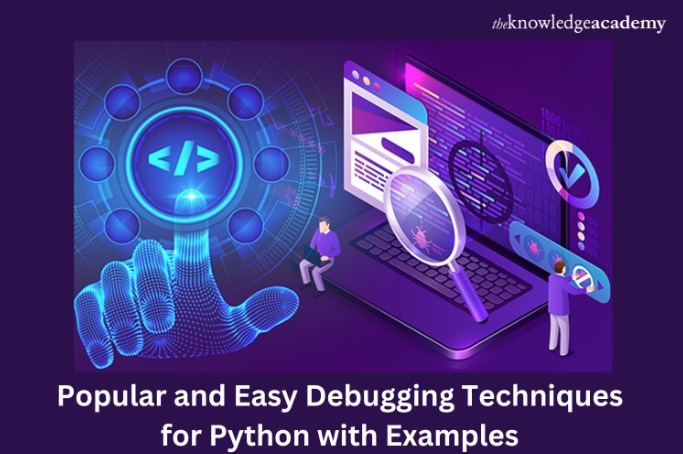
Python is a multipurpose programming language that is easy to learn and use. Python code will inevitably contain mistakes or bugs, especially as your projects get more complicated. To ensure their code functions as intended, every Python developer must be proficient in debugging. With the right Programming Training especially in Python Programming, you can refine your debugging techniques and avoid common pitfalls.
This blog will examine efficient techniques for debugging in Python with examples.
Table of Contents
- Why is Debugging Important?
- Common Types of Python Errors
- Debugging Techniques in Python
- Conclusion
Why is Debugging Important?
Debugging is a way to find and correct defects in a particular programme, known as bugs. If a programme has bugs, it may stop running, yield the incorrect output, or simply not run. Debugging allows developers to find out why these problems occur and then work on solving them.
Debugging improves your code and assures your programme works as intended. It also saves time in the long run by letting you target problems earlier in the development cycle, making the overall process easier to overcome.
Common Types of Python Errors
Before getting into debugging approaches, it’s useful to know what kinds of Python errors (also called bugs) are typically encountered:
1) Syntax Errors: These are caused by improper syntax that Python encounters, such as missing parenthesis or a colon. The interpreter will produce a syntax error because it cannot comprehend the code.
2) Runtime Errors: These happen when a programme is being executed. Two examples are division by zero and attempting to access an entry in a list that doesn’t exist.
3) Logical Errors: These are more difficult to debug because the software produces the wrong output even when no errors are thrown. Inaccurate presumptions or flawed logic in the code frequently lead to logical errors.
Debugging Techniques in Python
Let’s examine several efficient Python debugging techniques and explain how they operate.
1) Print Statements: Print statements are one of the easiest and most widely used debugging methods. They allow you to observe the values of your variables at different points in the programmes flow. Add them throughout your code.
Example:
In this example, the values of a, b, and result are shown using print statements at various stages in the function. This enables us to verify that the addition functions as intended and that the variables are correctly assigned.
Print statements can be helpful for basic debugging, but if you use them too often, they might clog your code. You might want to utilise more sophisticated debugging approaches for more complex programmes.
2) Using Try and Except Blocks: Gracious error handling is a crucial debugging component. Try and except blocks in Python, let you catch and manage exceptions without bringing down the whole application. This is particularly helpful for handling runtime faults that may arise under certain circumstances.
Example:
In this example, the except block detects the ZeroDivisionError if division by zero is attempted, and the try block tries to divide two numbers. This keeps the software from crashing and displays an informative error message.
3) Using the Python Debugger (pdb): The built-in debugger in Python, pdb, gives you a strong mechanism to step through your code line by line and examine variables at different points in time. With the pdb module, you may control the execution flow, check the values of variables, and set breakpoints.
Import the module, then call pdb.set_trace() to pause the execution at the desired place to begin debugging with pdb.
Example:
When you run this programme, an interactive debugging session will start at the line where pdb.set_trace() is located. Commands like n (next) let you advance to the next line; p (print) shows the value of a variable; and q (quit) allows you to exit the debugger.
4) Checking Stack Traces: When an error occurs in your programme, the Python interpreter provides a stack trace—a comprehensive summary of the error and the series of function calls that preceded it. Understanding stack traces is essential for determining the location and cause of the mistake.
Example:
The following stack trace will be produced when this code is run:
The stack trace demonstrates that the divide function, called by the calculate function, encountered the ZeroDivisionError when attempting to divide by zero. You can identify the exact location of the fault and begin troubleshooting, thereby tracking the stack trace.
5) Using Assertions: Using assertions can help you find logical mistakes in your application. Utilising an assert statement enables you to verify if a condition is true. Python stops the programme and raises an AssertionError if the condition is untrue.
Example:
Before determining the average, the assert statement in this example verifies that the list of grades is not empty. If the assertion fails, an error notice is shown, making it easier to find the problem immediately.
Conclusion
A crucial ability for any Python programmer is debugging. You may quickly find and correct errors in your code using more refined tools like the pdb debugger and basic strategies like printing statements and trying and accepting blocks. You will become a more confident developer, and your programming process will run more smoothly if you put these techniques into practice. To further enhance your debugging skills, check out free resources offered by The Knowledge Academy.